Chapter #3: How to Use and Declare Variables in Bash Scripts
In this guide, we will explore Bash scripting variables in depth, including how to use them effectively, along with essential types and techniques for working with variables.
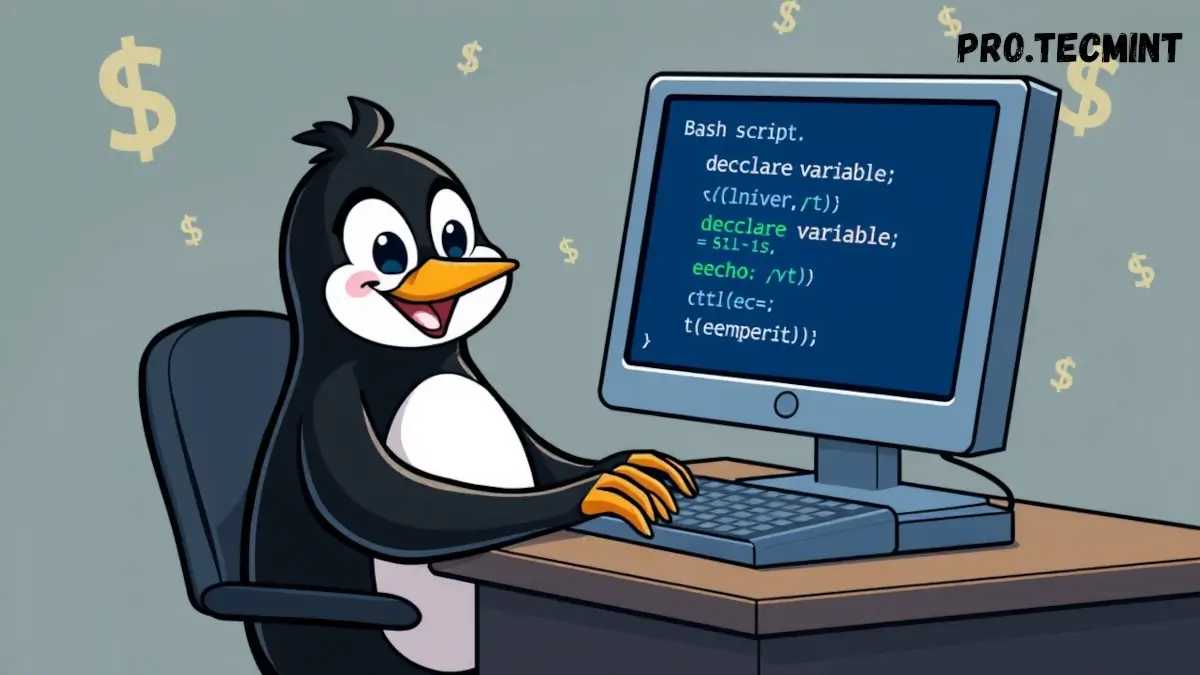
Shell scripting, like other scripting languages, has all the features and capabilities that make it a super powerful language for writing scripts.
One of the important concepts of programming is the use of variables. All programming languages use variables, with just a difference in how we define and use them.
In this guide, we will dive deep into variables in Bash scripting, how we can use them, and some types and techniques that we should know when dealing with variables.
What is a Variable?
You can think of a variable as a space or a box to store some information and use it later. This makes our code organized and reusable. You define a name and assign it a value, and whenever you need that value, you only need to type its name.
So, the takeaway from this is that each variable should have a name and a value:
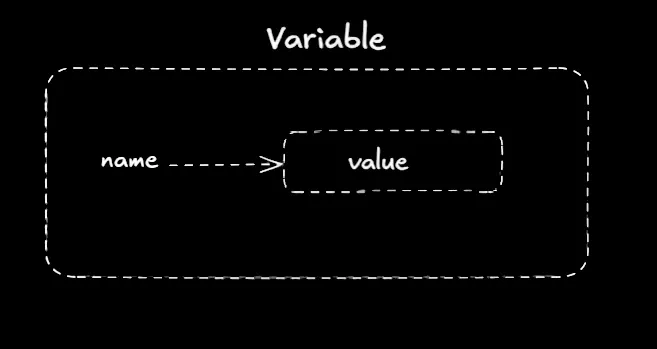
Declare a Variable in Bash
The variable declaration is the phase where we define the name and the value of the variable. We often call this operation initialization.
In Bash, to declare a variable, we use the following syntax:
myvar="pro.tecmint.com"
In the example above, we declare a variable with the name myvar
and the value pro.tecmint.com
, and in between them, we put the symbol =
without any spaces.
So, whenever we need the value pro.tecmint.com
, we only call the variable. What you also need to know is that the variable name should follow some rules that we need to keep in mind when creating a variable.