Chapter #8: How to Use for and while Loops in Bash Scripting
Learn how to use for loops, while loops, and the continue command in Bash to automate tasks, rename files, and write efficient shell scripts.
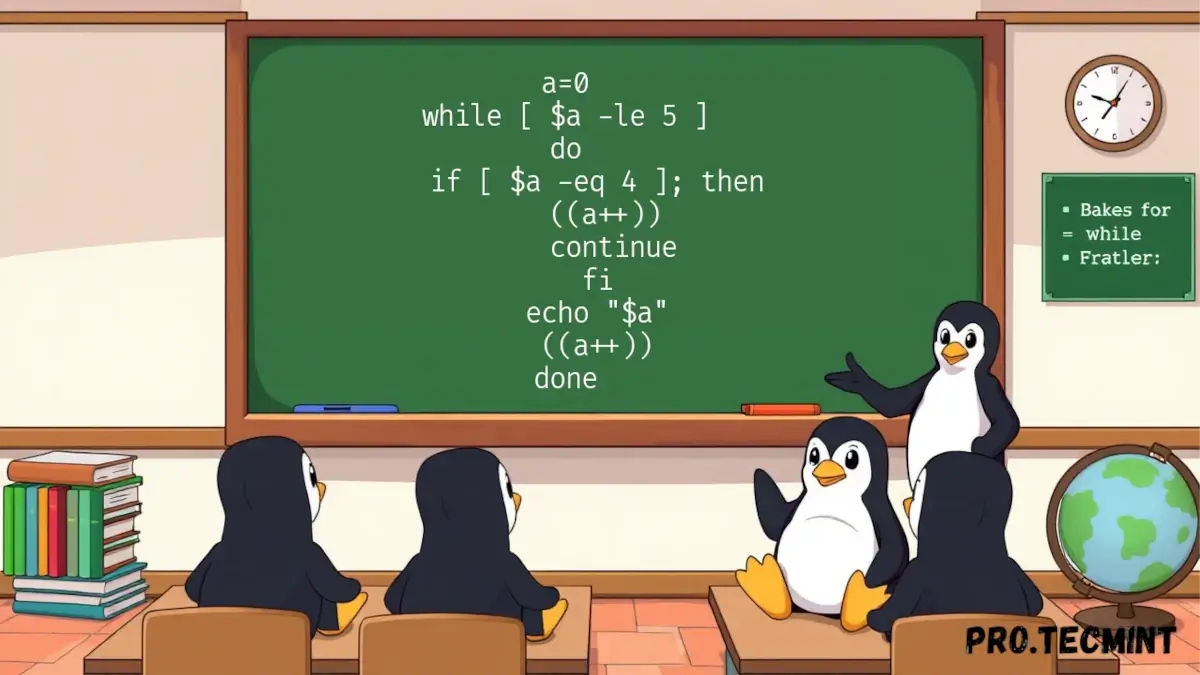
Until now, we’ve explored some game-changing concepts in our Bash scripting journey. As we said before, Bash is a fun scripting language that aims to make our automation and Linux controlling easier and more enjoyable.
Today, we're diving into one of the most powerful tools in any scripting language - loops. You might’ve heard them called iterations in other languages, and honestly, they’re everywhere. From checking files in a folder to running a command multiple times, loops help you repeat tasks without repeating yourself.
In this chapter, we’re going to learn how to write for
loops and while
loops in Bash. We’ll break it down with real-life examples, show you where they shine, and how to make your scripts way more efficient and smarter using them.
What are Loops
Loops let us perform a task over and over again, which is super handy when you don’t want to write the same line of code ten times. Unlike if statements (which only run something once if a condition is true), loops keep running a block of code until a certain condition is no longer true.
Just like in other programming languages, Bash gives us a few different types of loops to choose from. Each one has its own style, purpose, and best use case, but they all help us repeat tasks in a smarter way.