Chapter #12: How to Use Arrays in Bash for Server Monitoring and Backups
Learn how to create and use arrays in Bash, access elements, loop through them, and apply them in tasks like server checks and backups.
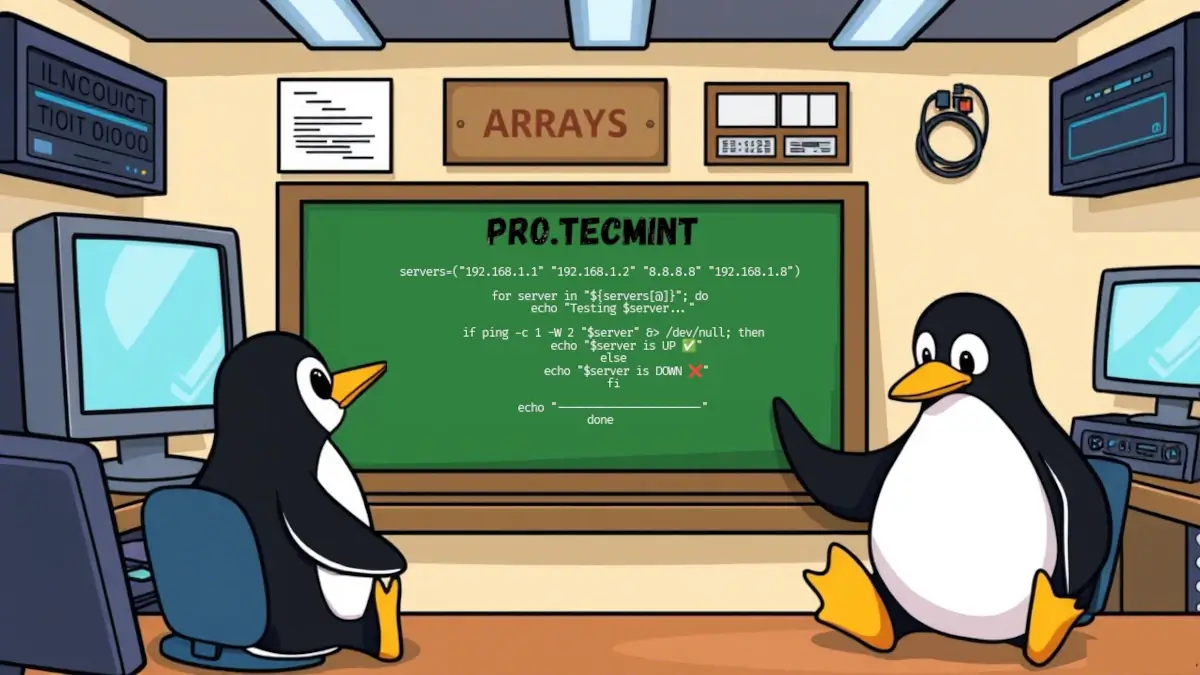
Till this point, we learned some interesting concepts and functionality that Bash scripts offer us in order to write minimalist and efficient scripts.
In today's lesson, we are going to explore another important concept in programming in general, which we call arrays
.
Just like other programming languages, Bash has what we call arrays
, which allow us to store multiple data in one single variable. If you remember the lesson on variables, we learned how to store a single value in a variable and then access it as we need it.
Arrays do the same thing, except instead of declaring multiple variables, we only have one that stores multiple values.
What are arrays?
Basically, an array is a variable that can store multiple values, and we can access them separately as we need them. The syntax is similar to what we use to declare a variable, with just a little difference.
To create an array in Bash, we use this syntax:
arr_name=("value1" "value2" ...)
Just like a variable, we start with the name followed by an equal sign, and inside parentheses, we put the values that we want, separated by spaces.
Let's take an example to make this simple:
names=("TecMint" "Bash" "Linux")
In this example, we declare an array called names
, and we put the values that we want. You get the idea, now instead of creating a variable for each value, we put them all in one variable, which we call an array.