Chapter #11: How to Use IFS and Read to Parse Files in Bash
In this article, you’ll learn how to split strings, skip empty lines, and read files line by line using Bash scripting.
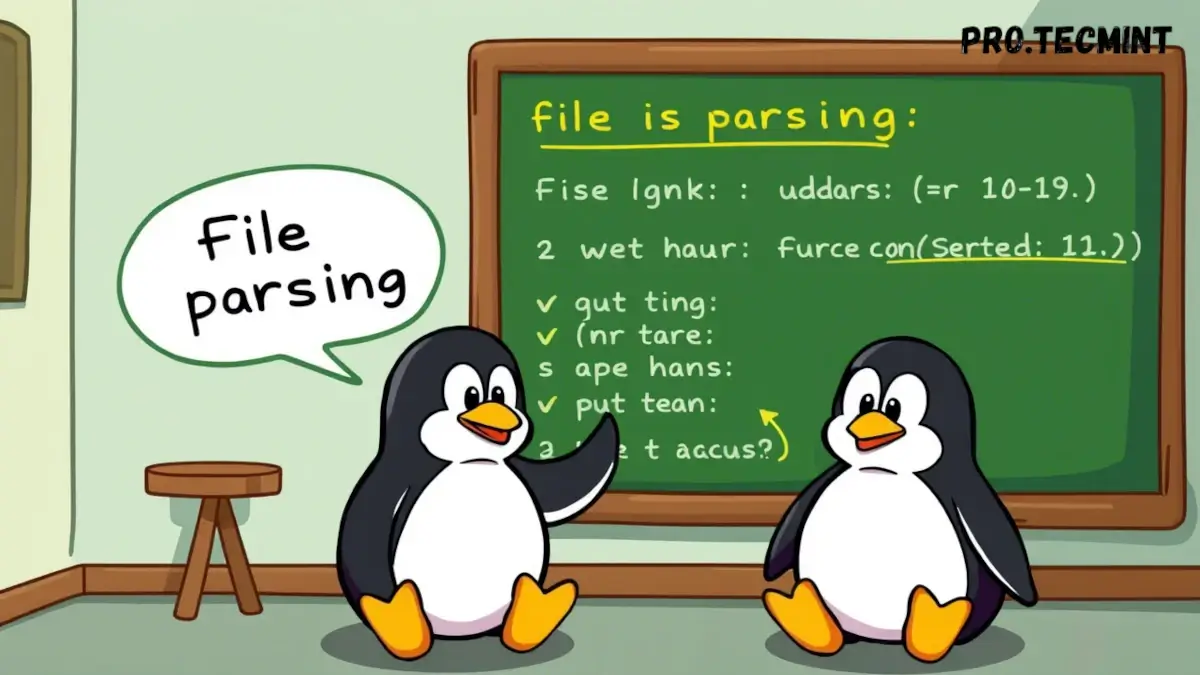
We’re picking up right where we left off - working with files in Bash. There’s a lot more you can do beyond just reading a file line by line.
File handling is a core part of Bash scripting. Whether you're automating tasks, building utilities, or managing system processes, you're going to be dealing with files all the time. So, knowing how to manipulate them efficiently is key.
We have separated this into multiple lessons so you can concentrate on each lesson and focus on what you learn from it.
In the last lesson, we learned how to read a file using the cat
command with some examples and simple Bash scripts that used a while
loop to read a text file line by line.
In this lesson, we will continue learning about file and text processing commands and techniques that you should know as a sysadmin.
Internal Field Separator (IFS)
IFS, or Internal Field Separator, is what Bash uses to decide how to split a string into words. By default, it splits on spaces, tabs, and newlines. But the cool part? You can change it to whatever you want.
I know you don’t understand this from this introduction, but when you do an example, you will understand what we mean here.