Chapter #10: How to Read Files in Bash with cat, While Loops & More
In this chapter, you'll learn the basics of reading and working with files in Bash using cat, loops, and redirection.
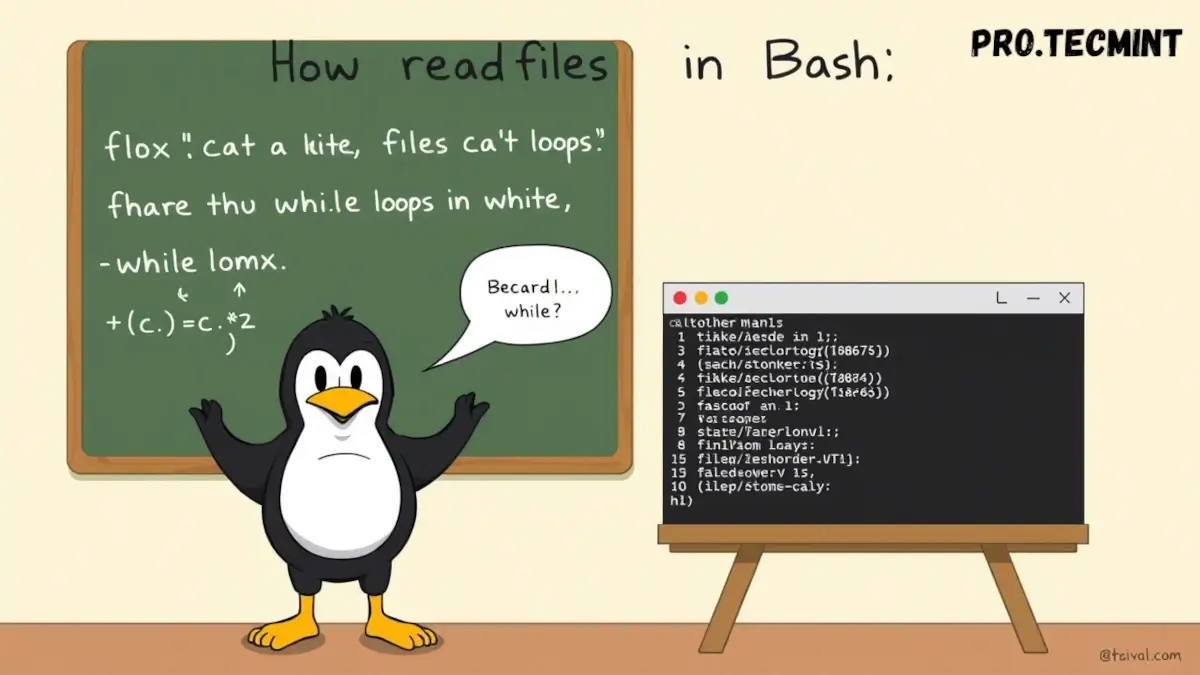
Till now, we've covered some key concepts in Bash, and our journey to mastering shell scripting continues.
Before diving deeper into Bash itself, we need to equip ourselves with an essential tool that will give us greater control over our system. You've likely heard the saying, "everything in Linux is a file," and it's true. To truly understand and gain more control over your system, it's crucial to grasp this idea.
In Bash scripting, working with files and directories is a fundamental skill. As a sysadmin, you'll need to master how to interact with them. A significant portion of your work in Linux will involve dealing with files and directories, whether you're reading from them or writing to them.
In this lesson, we'll explore how to handle files and directories with practical examples and real-world scenarios, so youβll be well-prepared to interact with them effectively.
Reading from a File
One of the essential operations you need to master is reading from files using Bash scripts. As a system administrator, or even as a developer, there will be times when you need to read from a file, either to view its contents or to pass that content to another tool or operation for further handling.
In Bash, there are several commands available for reading files. A quick reminder, which we might not have covered in the previous lesson: Bash commands fall into two main categories.