Chapter #9: How to Use Until Loops and Case Statements in Bash
In this chapter, we learn how to use until loops and case statements in Bash to make our scripts smarter and more flexible.
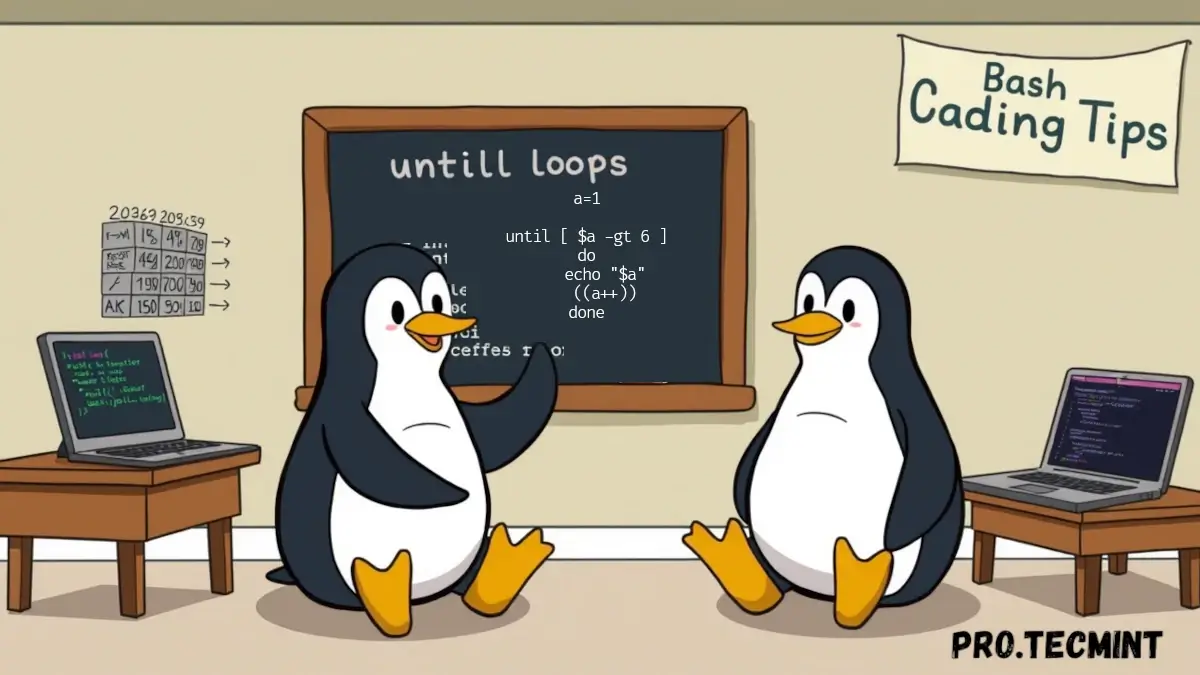
until
loops and case
statements in BashLoops are a powerful tool in Bash scripting that help make our scripts more efficient and dynamic. Instead of repeating the same block of code over and over, loops allow us to automate and make our code shorter, cleaner, and easier to maintain.
In the previous chapters, we learned how to use the for and while loops to repeat tasks. These loops are great for working with data, especially when dealing with files, directories, and other elements in a system.
But did you know there are other types of loops in Bash? In this chapter, weβll explore a couple of them: the until
loop and the case
statement. These are additional ways to make our scripts more flexible and powerful.
Letβs dive into these new loops and see how they work with examples!
Until Loop
The until
loop works similarly to the while
loop, but it runs in the opposite way.
In a while
loop, the code runs as long as the condition is true. But in an until
loop, the code runs until the condition becomes true. Once the condition is true, the loop stops.
The basic syntax of an until
loop looks like this:
until [ condition ]
do
command to execute
done
Just like the while
loop, we place the condition inside the brackets. The until
loop checks the condition and keeps running the code inside the loop until the condition becomes true. Once true, the loop exits.