Chapter #7: How to Use If Statements in Bash
In this article, we’ll help you level up your Bash scripting by adding decision-making power to your code with if statements.
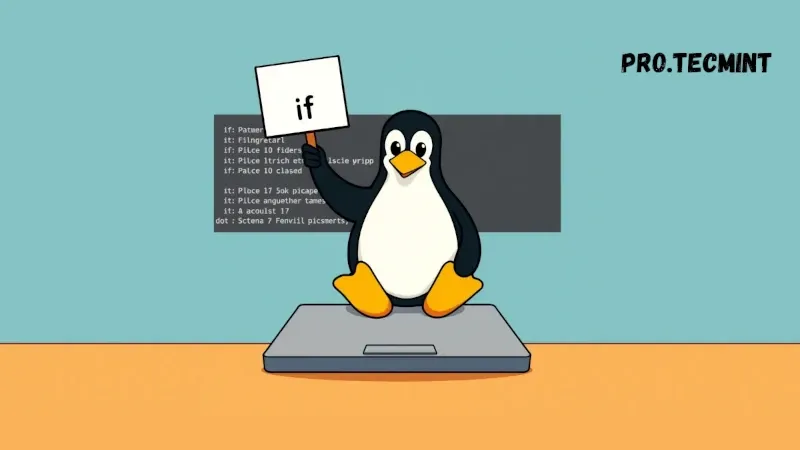
So far, we’ve been building a strong foundation in Bash scripting - creating scripts, using variables, handling user input, and playing with globbing and redirection. But up until now, our scripts have been kinda... one-track minded. They do something, and that’s it - no questions asked.
But what if we want our script to make decisions?
Welcome to the world of control flow!
In this chapter, we're stepping up our game by teaching your Bash scripts how to think. Well, not literally, but close enough! We'll introduce the mighty if
statement - your go-to tool when you want your script to behave differently based on conditions.
Whether you're checking a number, comparing strings, or evaluating the result of a command, if
statements let your script react and adapt. This is where automation really starts to feel smart.
Don’t worry if you’ve never seen an if
statement before. We’ll break it down super simply, just like we’ve done in every other chapter. And if you're coming from another programming language! You’ll feel right at home once you see the Bash syntax.
What is Control Flow?
Just like the name says, control flow is all about controlling how your script flows, or in simple terms, deciding what happens next based on certain conditions.
Up until now, our scripts have been doing one thing at a time, step by step. But with control flow, we can make our scripts smarter and more dynamic. Instead of always running the same instructions, our scripts can now react - doing one thing if a condition is true, and something else if it’s not.