Chapter #2: Set Up an AI Development Environment for Python on Linux
Learn how to set up an AI environment on Linux using Python, TensorFlow, and PyTorch with GPU support for faster model training.
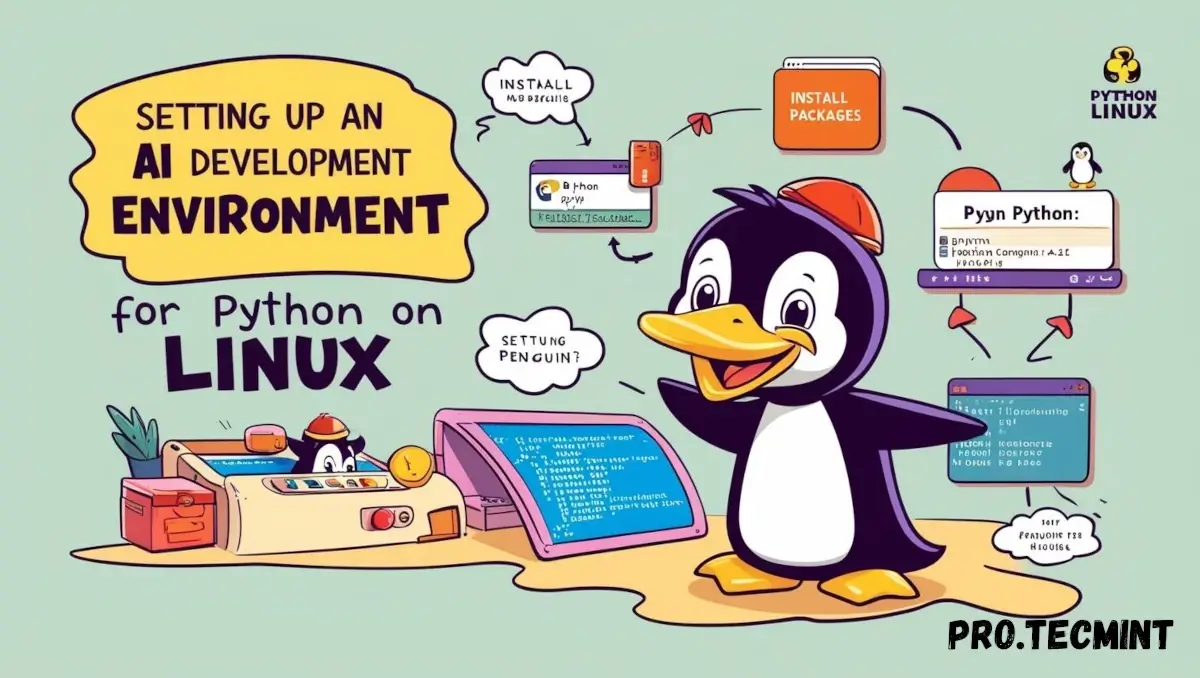
AI has seen incredible growth in the tech industry, especially in recent years, with the rise of large language models (LLMs) like OpenAI's GPT and DeepSeek, which have opened up a new and in-demand field for developers looking to start a career in AI by learning and exploring AI development processes.
Why Choose Linux over Windows for AI Development?
In general, Linux offers greater flexibility and control for development. Most AI tools are built and trained on Linux, making it the preferred environment for AI and machine learning.
It provides all the necessary packages and tools at your fingertips - just install them and start your journey. In contrast, setting up an AI development environment on Windows can be more challenging.
Linux has long been the preferred operating system for developers and data scientists working in AI and machine learning. Hereβs why:
- Open Source & Developer-Friendly: Linux offers more control, customization, and flexibility.
- AI Tools Compatibility: Most AI tools, frameworks, and libraries are developed and optimized for Linux.
- Better Package Management: Installing Python, pip, and other packages is much easier using tools like
apt
,yum
, ordnf
. - Efficient Resource Handling: Linux handles memory and CPU resources better than Windows in most development scenarios.
- Cloud-Ready: Major cloud providers (AWS, GCP, Azure) use Linux-based virtual machines by default.
Python is one of the most widely used programming languages in the field of AI, thanks to its simplicity and the vast ecosystem of libraries and frameworks it supports, including popular ones like PyTorch and TensorFlow.
In this guide, weβll walk you through the steps to set up a fully functional AI development environment on Linux, so you can start building and experimenting with AI tools right away.
What Is an AI Environment?
An AI environment refers to a collection of tools, libraries, and configurations installed on your system that enable you to develop, train, and test artificial intelligence and machine learning models.
Each programming language, especially Python, the most popular language in AI, comes with its own set of tools and frameworks that make up this development environment.
Setting up an AI environment involves installing and configuring everything you need to work on AI projects efficiently.